Last updated on February 12, 2021
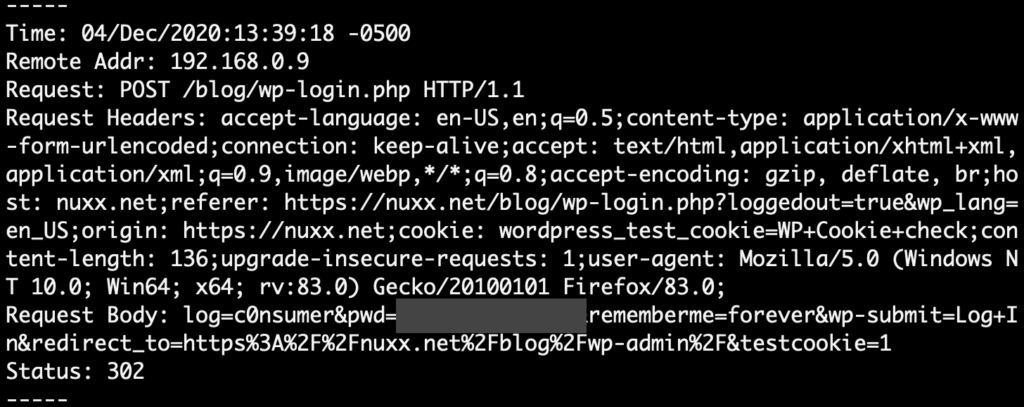
Consider the following situation: You have a web app from a vendor and during a security scan it crashes. The web app is running over HTTPS with your certificates, but neither the scanning tool nor web app offer sufficient logging to see exactly which request caused the crash.
Because you can’t decrypt HTTPS without access to a client key log file (or making a bunch of TLS changes), and the client is a security scanning tool, Wireshark is not an option to see the triggering request. Fiddler is also likely out, as that’d require the security scanner to trust a new root cert. So what can you do? Stick something else in the way to proxy the connection, logging all the requests!
Having access to the private certificates for the server this is quite easy: set up nginx as a proxy. The only wrinkle is that getting access to all of the request headers requires Lua, so you’ll need to ensure your nginx install supports this. On macOS this was easy using Homebrew to install nginx from denji’s GitHub repository (the default nginx doesn’t support Lua):
brew tap denji/nginx
brew install nginx-full --with-lua-module --with-set-misc-module
This configuration uses the web app’s certificates in nginx to proxy requests it receives to your main site, logging the client IP, request, headers, body, and request status to intercept.log
. Requests are broken out by line to make for easy visual reading. You may wish to move this all on to one line to make parsing easy:
events {
}
http {
log_format custom 'Time: $time_local'
'
'
'Remote Addr: $remote_addr'
'
'
'Request: $request'
'
'
'Request Headers: $request_headers'
'
'
'Body: $request_body'
'
'
'Status: $status'
'
'
'-----';
server {
listen 443 ssl;
server_name example.com;
access_log /path/to/intercept.log custom;
ssl_certificate /path/to/cert.pem;
ssl_certificate_key /path/to/privkey.pem;
location / {
proxy_pass https://example.com;
proxy_set_header Accept-Encoding '';
set_by_lua_block $request_headers {
local h = ngx.req.get_headers()
local request_headers_all = ""
for k, v in pairs(h) do
request_headers_all = request_headers_all .. ""..k..": "..v..";"
end
return request_headers_all
}
}
}
}
To put this in place, ensure that requests from the scanner go to nginx instead of the web app and then nginx will forward and log the requests. There are a few ways you could do this:
- Run nginx on the same server as the web app, move the web app to listen to another port for HTTPS, and set proxy_pass to the other port:
proxy_pass https://example.com:4430
- Run nginx on a new server, change the DNS records for the site to point to the new server, and point nginx to the old server by IP:
proxy_pass https://192.168.10.10
- If the scanner tool’s name resolution can be adjusted, such as via a HOSTS file or custom configuration, point it to the nginx proxy for the site name.
To test you can use a web browser on a client computer and a HOSTS file to point the original hostname nginx. To get the screenshot above I ran nginx on iMac running macOS, then in a Windows VM I changed the HOSTS file to map nuxx.net to the iMac’s IP. Firefox on the Windows VM then sent requests for nuxx.net to nginx on macOS which logged and proxied the requests out to the real nuxx.net.